
flow table final states, the state diagram and the asynchronous circuit schematic1 – Generally, a lawn mower has a mechanical interlock that prevents the engine start without a safety button being pressed before turning on the key. By replacing this mechanical part by an electronic equivalent, this safety mechanism becomes easier to manufacture, reducing the cost of the product.The lawn mower in question has a safety button B that must be pressed before the on / off switch C. When the safety button is pressed (that is, when it goes to level logic 1), an LED indicator L is lit; then, when switching C to ‘alloy’ (logic level 1), the motor will start (indicated by an output M at logic level 1). The engine will stop when it is switched to ‘shut down’ (logic level 0) or when the security key is released (logic level 0). The security button B it must be activated again (that is, a change from 0 to 1) before restarting the engine. Note that the system to be developed should guarantee that the engine will only be started when B is pressed before turning the key on. Include in your project the primitive flow table, the flow table final states, the state diagram and the asynchronous circuit schematic. Detail the decisions of project
class Player:
“””
Represents a player of the FocusGame. Has name and color attributes, as well as attributes to count captured and
reserved pieces. Has getter methods for all attributes, as well as methods to add/decrement reserved pieces and add
captured pieces.
Player objects are initialized when a FocusGame object is initialized. Initialization of a FocusGame object requires
name and color piece parameters for two players, and these parameters are then used to initialize two Player objects.
Player objects are stored in and used by FocusGame objects, so that the FocusGame object can utilize the Player’s color,
reserved, and captured attributes when doing game logic that checks for wins, makes reserve moves, puts pieces into
reserve and capture, checks for correct color on top of the stack, etc.
“””
def __init__(self, name, color):
self._name = name.upper()
self._color = color.upper()
self._captured = 0
self._reserved = 0
def get_color(self):
return self._color
def get_name(self):
return self._name
def get_captured_pieces(self):
return self._captured
def get_reserved_pieces(self):
return self._reserved
def decrement_reserved_pieces(self):
self._reserved -= 1
def add_reserved_piece(self):
self._reserved += 1
def add_captured_piece(self):
self._captured += 1
class Space:
“””
Represents a space on the FocusGame board. Multiple pieces on the space are represented by a list (the stack attribute),
with lowest piece at index 0, and top piece at highest index.
Has methods for adding a piece, removing pieces from top and bottom, getting length, and getting the stack itself.
Space objects are initialized in a 6×6 list of lists (to represent the board) when a FocusGame object is initialized.
“””
def __init__(self, starting_piece):
“””Initializes a Space object. If given a starting piece, adds that starting piece to the stack.”””
if not starting_piece:
self._stack = []
else:
self._stack = [starting_piece.upper()]
def add_piece(self, piece):
“””
Adds piece to top of the stack (end of the stack list).
:param piece: the color of the piece to add (string)
:return: None
“””
self._stack.append(piece)
def pop_top(self):
“””
Removes the piece on the top of the stack (at the end of the stack list) and returns it.
:return: piece (string)
“””
if not self.is_empty():
return self._stack.pop(len(self._stack) – 1)
return None
def get_top(self):
“””
Returns the piece on the top of the stack without removing it.
Used for checking the color of the piece on the top of the stack.
:return: piece (string)
“””
if not self.is_empty():
return self._stack[len(self._stack) – 1]
return None
def is_empty(self):
if len(self._stack) == 0:
return True
return False
def remove_pieces_from_bottom(self):
“””
If the stack has over 5 pieces in it, removes pieces from the bottom of the stack (beginning of the stack list)
until the stack has only 5 pieces in it. Returns a list of all pieces removed and stores it in extra_pieces.
Used when capturing and reserving pieces after a move has been made.
:return: extra_pieces (list of pieces removed)
“””
extra_pieces = []
if len(self._stack) > 5:
extra_qty = len(self._stack) – 5
for i in range(extra_qty):
extra_pieces.append(self._stack.pop(0))
return extra_pieces
def remove_pieces_from_top(self, num_pieces):
“””
Removes a specified number of pieces from the top of the stack, and returns them in a list.
Used when making a single or multiple move.
Returns a list of pieces in reverse order of how they’ll be placed on the destination space,
i.e. the top piece of the stack of moving pieces is at index 0 in piece_stack.
:param num_pieces: number of pieces to remove from top (int)
:return: piece_stack (list of pieces removed)
“””
piece_stack = []
for i in range(num_pieces):
piece_stack.append(self.pop_top())
return piece_stack
def get_length(self):
return len(self._stack)
def get_stack(self):
return self._stack
class FocusGame:
“””
Represents the Focus game. Has attributes for two players, each of which are a Player object. Has an attribute to
track whose turn it is, and a “board” attribute that uses a list of lists (of Space objects) to represent the board.
The main methods used for gameplay are the move_piece and reserved_move methods. The make_move, change_turn, and
reserve_and_capture methods perform subtasks for the main gameplay methods. is_win and check_reserve_and_capture check
for winning condition and reserve/capture condition, respectively.
The find_player_by_name method is used to identify the Player object making the current move, and the get_space
method is used to identify the Space objects at the move’s origin/destination.
Several methods validate user input for the main gameplay methods, such as is_correct_turn, is_valid_position,
is_valid_location, and is_valid_piece_num.
Additionally, there are methods to show current statuses within the game, such as show_pieces, show_reserve, and show_captured.
“””
def __init__(self, player_a, player_b):
self._player_a = Player(player_a[0].upper(), player_a[1].upper())
self._player_b = Player(player_b[0].upper(), player_b[1].upper())
self._current_turn = None # will be Player object
self._board = [[Space(self._player_a.get_color()), Space(self._player_a.get_color()), Space(self._player_b.get_color()), Space(self._player_b.get_color()), Space(self._player_a.get_color()), Space(self._player_a.get_color())],
[Space(self._player_b.get_color()), Space(self._player_b.get_color()), Space(self._player_a.get_color()), Space(self._player_a.get_color()), Space(self._player_b.get_color()), Space(self._player_b.get_color())],
[Space(self._player_a.get_color()), Space(self._player_a.get_color()), Space(self._player_b.get_color()), Space(self._player_b.get_color()), Space(self._player_a.get_color()), Space(self._player_a.get_color())],
[Space(self._player_b.get_color()), Space(self._player_b.get_color()), Space(self._player_a.get_color()), Space(self._player_a.get_color()), Space(self._player_b.get_color()), Space(self._player_b.get_color())],
[Space(self._player_a.get_color()), Space(self._player_a.get_color()), Space(self._player_b.get_color()), Space(self._player_b.get_color()), Space(self._player_a.get_color()), Space(self._player_a.get_color())],
[Space(self._player_b.get_color()), Space(self._player_b.get_color()), Space(self._player_a.get_color()), Space(self._player_a.get_color()), Space(self._player_b.get_color()), Space(self._player_b.get_color())]]
def move_piece(self, player_name, orig_coord, dest_coord, num_pieces):
“””
Calls methods to check for correct player turn, valid origin and destination locations, and valid number of pieces.
If everything is valid, calls methods to make the pieces move from origin to destination, check for need to
reserve and capture, check for a win, and change current turn.
“””
player = self.find_player_by_name(player_name)
if not self.is_correct_turn(player):
return “Not your turn”
if not self.is_valid_location(player, orig_coord, dest_coord, num_pieces):
return “Invalid location”
orig = self.get_space(orig_coord)
if not self.is_valid_piece_num(orig, num_pieces):
return “Invalid number of pieces”
dest = self.get_space(dest_coord)
self.make_move(orig, dest, num_pieces)
self.check_reserve_and_capture(dest, player)
if self.is_win(player):
return player_name + ” Wins”
self.change_turn(player)
return “Successfully moved”
def show_pieces(self, position):
if not self.is_valid_position(position):
return None
return self._board[position[0]][position[1]].get_stack()
def show_reserve(self, player_name):
player = self.find_player_by_name(player_name)
if player:
return player.get_reserved_pieces()
return
def show_captured(self, player_name):
player = self.find_player_by_name(player_name)
if player:
return player.get_captured_pieces()
return
def reserved_move(self, player_name, position):
# check if valid position
if not self.is_valid_position(position):
return
# check if player has pieces in reserve
player = self.find_player_by_name(player_name)
if not player or player.get_reserved_pieces() == 0:
return “No pieces in reserve”
# add a reserved piece onto the destination stack, and subtract one from player’s reserved pieces
dest = self.get_space(position)
dest.add_piece(player.get_color())
player.decrement_reserved_pieces()
# check for reserve and capture, check for win condition, change turn
self.check_reserve_and_capture(dest, player)
if self.is_win(player):
return player_name + ” Wins”
self.change_turn(player)
def is_valid_position(self, position):
if position[0] < 0 or position[1] < 0 or position[1] > 5 or position[1] > 5:
return False
return True
def get_space(self, position):
return self._board[position[0]][position[1]]
def find_player_by_name(self, name):
if name.upper() == self._player_a.get_name():
return self._player_a
elif name.upper() == self._player_b.get_name():
return self._player_b
return None
def change_turn(self, current_player):
if current_player == self._player_a:
self._current_turn = self._player_b
else:
self._current_turn = self._player_a
def check_reserve_and_capture(self, dest, player):
if dest.get_length() > 5:
self.reserve_and_capture_pieces(dest, player)
def reserve_and_capture_pieces(self, dest, player):
extra_pieces = dest.remove_pieces_from_bottom()
for piece in extra_pieces:
if piece == player.get_color():
player.add_reserved_piece()
else:
player.add_captured_piece()
def is_win(self, player):
if player.get_captured_pieces() >= 6:
return True
return False
def make_move(self, orig, dest, num_pieces):
pieces_moved = orig.remove_pieces_from_top(num_pieces)
for i in range(len(pieces_moved)):
dest.add_piece(pieces_moved[len(pieces_moved) – 1 – i])
def is_correct_turn(self, player):
if self._current_turn is not None and player != self._current_turn:
return False
return True
def is_valid_location(self, player, orig_coord, dest_coord, num_pieces):
# if invalid origin or destination coordinates
if not self.is_valid_position(orig_coord) or not self.is_valid_position(dest_coord):
return False
# if orig is a stack with their piece not on top
orig = self.get_space(orig_coord)
if orig.get_top() != player.get_color():
return False
# invalid location if attempt to move diagonally
row_diff = dest_coord[0] – orig_coord[0]
col_diff = dest_coord[1] – orig_coord[1]
if row_diff != 0 and col_diff != 0:
return False
# invalid location if spaces moved is not equal to number of pieces being moved
if row_diff == 0:
spaces_moved = abs(col_diff)
else:
spaces_moved = abs(row_diff)
if num_pieces != spaces_moved:
return False
return True
def is_valid_piece_num(self, orig, num_pieces):
if num_pieces > orig.get_length():
return False
return True
Get professional assignment help cheaply
Are you busy and do not have time to handle your assignment? Are you scared that your paper will not make the grade? Do you have responsibilities that may hinder you from turning in your assignment on time? Are you tired and can barely handle your assignment? Are your grades inconsistent?
Whichever your reason may is, it is valid! You can get professional academic help from our service at affordable rates. We have a team of professional academic writers who can handle all your assignments.
Our essay writers are graduates with diplomas, bachelor, masters, Ph.D., and doctorate degrees in various subjects. The minimum requirement to be an essay writer with our essay writing service is to have a college diploma. When assigning your order, we match the paper subject with the area of specialization of the writer.
Why choose our academic writing service?
Plagiarism free papers
Timely delivery
Any deadline
Skilled, Experienced Native English Writers
Subject-relevant academic writer
Adherence to paper instructions
Ability to tackle bulk assignments
Reasonable prices
24/7 Customer Support
Get superb grades consistently
Get Professional Assignment Help Cheaply
Are you busy and do not have time to handle your assignment? Are you scared that your paper will not make the grade? Do you have responsibilities that may hinder you from turning in your assignment on time? Are you tired and can barely handle your assignment? Are your grades inconsistent?
Whichever your reason may is, it is valid! You can get professional academic help from our service at affordable rates. We have a team of professional academic writers who can handle all your assignments.
Our essay writers are graduates with diplomas, bachelor’s, masters, Ph.D., and doctorate degrees in various subjects. The minimum requirement to be an essay writer with our essay writing service is to have a college diploma. When assigning your order, we match the paper subject with the area of specialization of the writer.
Why Choose Our Academic Writing Service?
Plagiarism free papers
Timely delivery
Any deadline
Skilled, Experienced Native English Writers
Subject-relevant academic writer
Adherence to paper instructions
Ability to tackle bulk assignments
Reasonable prices
24/7 Customer Support
Get superb grades consistently
How It Works
1. Place an order
You fill all the paper instructions in the order form. Make sure you include all the helpful materials so that our academic writers can deliver the perfect paper. It will also help to eliminate unnecessary revisions.
2. Pay for the order
Proceed to pay for the paper so that it can be assigned to one of our expert academic writers. The paper subject is matched with the writer’s area of specialization.
3. Track the progress
You communicate with the writer and know about the progress of the paper. The client can ask the writer for drafts of the paper. The client can upload extra material and include additional instructions from the lecturer. Receive a paper.
4. Download the paper
The paper is sent to your email and uploaded to your personal account. You also get a plagiarism report attached to your paper.
PLACE THIS ORDER OR A SIMILAR ORDER WITH Essay fount TODAY AND GET AN AMAZING DISCOUNT
The post asynchronous circuit schematic appeared first on Essay fount.
What Students Are Saying About Us
.......... Customer ID: 12*** | Rating: ⭐⭐⭐⭐⭐"Honestly, I was afraid to send my paper to you, but you proved you are a trustworthy service. My essay was done in less than a day, and I received a brilliant piece. I didn’t even believe it was my essay at first 🙂 Great job, thank you!"
.......... Customer ID: 11***| Rating: ⭐⭐⭐⭐⭐
"This company is the best there is. They saved me so many times, I cannot even keep count. Now I recommend it to all my friends, and none of them have complained about it. The writers here are excellent."
"Order a custom Paper on Similar Assignment at essayfount.com! No Plagiarism! Enjoy 20% Discount!"
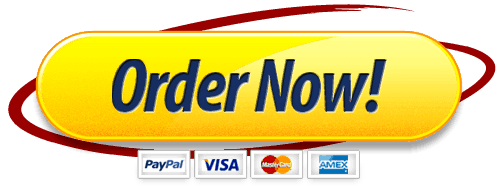