
include
include
include
include
include
include
//Global variablesint Max_Num = 0;int* Prime = NULL;int N =0;int bufferSize = 0;int bufferIn =0;int bufferOut = 0;int count = 0;//File to handle large inputsFILE* file;
//Semaphoresem_t empty;sem_t full;//Mutexpthread_mutex_t Lock_Prime;
void *primeThread();void *printPrime();
int main(){char *x = NULL;char arr[32];//Initialise mutexpthread_mutex_init(&Lock_Prime, NULL);//Create a text file to store outputfile = fopen(“output.txt”, “w”);printf(“Enter an integer: ” );//Get the rangex = fgets(arr, 32, stdin);Max_Num = atoi(arr);
//Buffer sizebufferSize = 0.4 * Max_Num;//Initialise the bufferPrime = (int)malloc(bufferSizesizeof(int));sem_init(&empty,0,bufferSize);sem_init(&full,0,0);
int* value = (int)malloc((Max_Num/2)sizeof(int));
//Count the prime using Sieve of Eratosthenes algorithmint value1[Max_Num+1];for(int i =0;i<Max_Num+1;i++)value1[i]=1;
for (int p=2; p*p<=Max_Num; p++)
{
if (value1[p] == 1)
{
for (int i=p*p; i<=Max_Num; i += p)
{
value1[i] = 0;
}
}
}
for (int p=2; p<=Max_Num; p++)
{ if (value1[p])
{
value[count] = p;
count++;
}
}
printf(“nTotal number of Prime numbers: %dn”,count);printf(“nPrime Numbers: “);//Initialise prime and print threadpthread_t* prime = (pthread_t)malloc((count)sizeof(pthread_t));pthread_t* print = (pthread_t)malloc((count)sizeof(pthread_t));//Create the threadsfor(int i=0; i<count;i++){pthread_create(&prime[i], NULL, (void )primeThread, (void)&value[i]);pthread_create(&print[i], NULL, (void )printPrime, (void)&i);}//Join the threadsfor(int i = 0; i < count; i++) {pthread_join(prime[i], NULL);pthread_join(print[i], NULL);}return 0;
}
void *primeThread(int *value){int num = *value;//Lock the spacesem_wait(&empty);pthread_mutex_lock(&Lock_Prime);//Store the value in the bufferPrime[bufferIn]= num;bufferIn = (bufferIn + 1)%bufferSize;//Unlock the spacepthread_mutex_unlock(&Lock_Prime);sem_post(&full);return NULL;}
void* printPrime(int *id){//Lock the spacesem_wait(&full);pthread_mutex_lock(&Lock_Prime);//Print to filefprintf(file, “%d “, Prime[bufferOut]);//Print the value to the bufferprintf(“%d “,Prime[bufferOut]);bufferOut = (bufferOut + 1)%bufferSize;//Unlock the spacepthread_mutex_unlock(&Lock_Prime);sem_post(&empty);
return NULL;
}
Review: Synchronization TechniquesBusy Waiting◦ Locks◦ Strict Alternation◦ Peterson’s SolutionSleep & Wakeup◦ Mutexes◦ Semaphores3Hardware Solutions• Disable Interrupts• TSL InstructionsSoftware SolutionsSynchronization techniques sofar:Main Issue:◦ Incredibly inefficient“Busy” waiting requires CPU time torepeatedly check if the resource isavailable◦ Unless maximum responsivity isrequired (User interfaces), thereare too many checks.4Mutex : Mutual ExclusionMutex is a tool that is used to ensure mutual exclusion on resources, that is one resources at time.POSIX API provides mutex interface that you can use in your programs as part of the pthread.h library.Functions Include: int pthread_mutex_init(pthread_mutex_t *restrict mutex, const pthread_mutexattr_t *restrictattr);initialize a mutex to be used within your program int pthread_mutex_lock(pthread_mutex_t *mutex)Used to lock access to a resources before accessing the resource. int pthread_mutex_unlock(pthread_mutex_t *mutex);Unlock access to resource after usage with it is done to allow access to other threads. int pthread_mutex_destroy(pthread_mutex_t *mutex);Destroy the mutex when program and synchronization is no longer needed.Example:6Can you find the critical section in this code?Example: MUTEX7How many threads can I add and expectcorrect response?Semaphores8 While Mutex ensures that only one thread access a resources at atime, semaphore acts as a traffic signal.Semaphores are a way to organize the order of access to a sharedresources.SemaphoresInstead of a binary system (0 or 1), use an integer◦ Max number of items within a shared resource,◦ If a buffer’s size is 100 bytes and 1 byte per write/read, then 100 itemsSemaphore operations are atomic.◦ Uses TSL instructions to ensure one process access the semaphore value at a time.9SemaphoresSemaphore operations are atomic.◦ Meaning that they can not be interrupted while in operation.Operations:wait()If (semaphore == 0): sleepelse: decrement semaphorepost()Increment semaphore10Producer-consumer problemProducer-consumer problem with semaphores:◦ The producer process generates an element and stores it in a shared resource(for example buffer).◦ The producer will signal that there is an element to consume (post operation).◦ The consumer process waits for a signal from the producer before it can consumean element. (wait operation)◦ With these, many processes can access the buffer11Producer-consumer problemwith semaphores◦ buffer status can range from 0-100 (empty to full)◦ Two semaphores are used to manage the buffer◦ To the producer (emptyCount)◦ To the consumer (fillCount)12Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)13//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 100fillCount: 0Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)14//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 100fillCount: 0fillCount == 0:sleep!Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)15//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 100fillCount: 0Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)16//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 99fillCount: 0Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)17//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 99fillCount: 1Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)18//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 99fillCount: 1fillCount == 1:wake!Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)19//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 99fillCount: 0Semaphores//Consumerloop forever:wait(fillCount)consume(Buffer)post(emptyCount)20//Producerloop forever:wait(emptyCount)write_to(Buffer)post(fillCount)emptyCount: 100fillCount: 0POSIX Semaphores POSIX provides a construct to organize access to shared resources to ensure correctsequence of access.Functions of semaphore is defined in semaphore.h int sem_init(sem_t *sem, int pshared, unsigned int value);initialize a semaphore with a value.int sem_wait(sem_t *sem);Wait for a post operation in the semphore (or don’t wait if semphore value is non-zero)int sem_post(sem_t *sem);Increment the value of the semaphore and wakeup one waiting thread .int sem_destroy(sem_t *sem);destroys the semaphore; no threads should be waiting on the semaphore if its destruction is tosucceed.
Synchronization With the ability to more than one process and threads executeconcurrently, problems such as synchronization and race conditionsoccur. More than one process or thread tries to access and write/read a resource atthe same time. This causes data to be inconsistent, depending on the order of execution.Lock Variablesshared var balance;shared var lock = 0if (withdrawChoice):while (lock == 1); //do nothingcrit_section();3crit_section():lock = 1if (withdrawAmount > balance):reject()else:balance -= withdrawAmountdispense(withdrawAmount)lock = 0Does this provide mutual exclusion?A lock that uses Busy-waiting is called a spin lockLock Variablesshared var balance;shared var lock = 0if (withdrawChoice):while (lock == 1); //do nothingcrit_section()4crit_section():lock = 1if (withdrawAmount > balance):reject()else:balance -= withdrawAmountdispense(withdrawAmount)lock = 0ProcessAProcessB
Only one process inside its critical section at a time
No assumptions made about speeds or number of CPUs
No Processes outside of its critical sections may block others
Processes should not wait forever to enter a critical sectionIssue with Lock Variables:Race condition is not fixedBoth processes get into busy wait “simultaneously“Both processes see that lock == 0Both processes go into crit_section()◦ First process sets lock from 0 to 1◦ Second process sets lock from 1 to 1 (!)Both processes check balance before one withdraws(withdrawAmount > balance) is not checked properlyEnd up with No control over race conditions.5Busy Waiting: Strict AlternationProcess a◦ 0 == locked◦ 1 == unlockedProcess b◦ 1 == locked◦ 0== unlocked6• Use turns: like locks, but instead of both processes using the same values, theyuse two different and opposite mapping.Busy Waiting: Strict Alternation7• Consider the following situation, Process (a) enters and leaves its critical section, thus turningturn to 1 Now process (a) and (b) in noncritical sections. Consider process (a) finishes quickly and loops back to checkturn, while process (b) still busy in noncritical section. Process (a) must wait till process (b) get it turn.What do you think of this solution?
Only one process inside its critical section at a time
No assumptions made about speeds or number of CPUs
No Processes outside of its critical sections may block others
Processes should not wait forever to enter a critical sectionBusy Waiting: Strict AlternationCan work with >2 processes (loop turn variables value from 0 to n-1)Works well if each process enters critical sections evenlyAlthough this solution fixes race conditions, it only work if two processesalternate turns to use a resource, which is not a real candidate for ageneral solution.Inefficient in reality:◦ Faster processes must wait on slower ones◦ Processes can’t take two turns back-to-backeven if the resource is available (hence: strictalternation)8Busy Waiting: Peterson’s Solution9Combines the idea of turnswith lock variables.Guarantees mutual exclusionTwo variables◦ int turn: who is up next◦ Boolean array flag[2]◦ Is set for the process trying to enter thecritical sectionshared var turnshared var flag[2]//P0 wants to enter critical sectionflag[0] = trueturn = 1 //process 0 turnwhile (flag[1] && turn == 1); //busy waitingCritical_section(); //execute critical section…flag[0] = false //Done with critical sectionPeterson’s Solution10shared var turnshared var flag[2]//P0: try to enter critical sectionflag[0] = trueturn = 1while (flag[1] && turn == 1)://busy waiting//execute critical sectionflag[0] = falseshared var turnshared var flag[2]//P1: try to enter critical sectionflag[1] = trueturn = 0while (flag[0] && turn == 0)://busy waiting//execute critical sectionflag[1] = falsePeterman’s SolutionProcess 0 wants to enter its critical section:◦ Set flag[0] = 1◦ Set turn = 1◦ If the other process is also interested (if flag[1] == 1):◦ Check turn; if (turn == 1) then busy wait until other is done◦ Note: turn has the opposite meaning in Peterman’s solution; if you are thelast to set the turn variable, then you are NOT up11
Get professional assignment help cheaply
Are you busy and do not have time to handle your assignment? Are you scared that your paper will not make the grade? Do you have responsibilities that may hinder you from turning in your assignment on time? Are you tired and can barely handle your assignment? Are your grades inconsistent?
Whichever your reason may is, it is valid! You can get professional academic help from our service at affordable rates. We have a team of professional academic writers who can handle all your assignments.
Our essay writers are graduates with diplomas, bachelor, masters, Ph.D., and doctorate degrees in various subjects. The minimum requirement to be an essay writer with our essay writing service is to have a college diploma. When assigning your order, we match the paper subject with the area of specialization of the writer.
Why choose our academic writing service?
Plagiarism free papers
Timely delivery
Any deadline
Skilled, Experienced Native English Writers
Subject-relevant academic writer
Adherence to paper instructions
Ability to tackle bulk assignments
Reasonable prices
24/7 Customer Support
Get superb grades consistently
Get Professional Assignment Help Cheaply
Are you busy and do not have time to handle your assignment? Are you scared that your paper will not make the grade? Do you have responsibilities that may hinder you from turning in your assignment on time? Are you tired and can barely handle your assignment? Are your grades inconsistent?
Whichever your reason may is, it is valid! You can get professional academic help from our service at affordable rates. We have a team of professional academic writers who can handle all your assignments.
Our essay writers are graduates with diplomas, bachelor’s, masters, Ph.D., and doctorate degrees in various subjects. The minimum requirement to be an essay writer with our essay writing service is to have a college diploma. When assigning your order, we match the paper subject with the area of specialization of the writer.
Why Choose Our Academic Writing Service?
Plagiarism free papers
Timely delivery
Any deadline
Skilled, Experienced Native English Writers
Subject-relevant academic writer
Adherence to paper instructions
Ability to tackle bulk assignments
Reasonable prices
24/7 Customer Support
Get superb grades consistently
How It Works
1. Place an order
You fill all the paper instructions in the order form. Make sure you include all the helpful materials so that our academic writers can deliver the perfect paper. It will also help to eliminate unnecessary revisions.
2. Pay for the order
Proceed to pay for the paper so that it can be assigned to one of our expert academic writers. The paper subject is matched with the writer’s area of specialization.
3. Track the progress
You communicate with the writer and know about the progress of the paper. The client can ask the writer for drafts of the paper. The client can upload extra material and include additional instructions from the lecturer. Receive a paper.
4. Download the paper
The paper is sent to your email and uploaded to your personal account. You also get a plagiarism report attached to your paper.
PLACE THIS ORDER OR A SIMILAR ORDER WITH Essay fount TODAY AND GET AN AMAZING DISCOUNT
The post essay on Eratosthenes algorithm appeared first on Essay fount.
What Students Are Saying About Us
.......... Customer ID: 12*** | Rating: ⭐⭐⭐⭐⭐"Honestly, I was afraid to send my paper to you, but you proved you are a trustworthy service. My essay was done in less than a day, and I received a brilliant piece. I didn’t even believe it was my essay at first 🙂 Great job, thank you!"
.......... Customer ID: 11***| Rating: ⭐⭐⭐⭐⭐
"This company is the best there is. They saved me so many times, I cannot even keep count. Now I recommend it to all my friends, and none of them have complained about it. The writers here are excellent."
"Order a custom Paper on Similar Assignment at essayfount.com! No Plagiarism! Enjoy 20% Discount!"
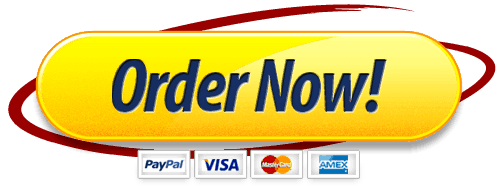