
Discussion Forum economics
Assignment 5: Process Scheduler SimulationCSci 430: Introduction to Operating SystemsFall 2020OverviewIn this assignment you will be implementing some of the process schedulers that are discussed in our textbookchapter 9. As with the previous assignment, your first task will be to implement some of the missing pieces of thegeneral process scheduling simulator framework, that supports plugging in different job scheduling policies to makedispatching decisions. The simulator will take a table of job arrival information along with the service time (runtime) of the jobs, just as is used in our textbook for discussing the various processes scheduling policies. We will besimulating a single CPU system with this process scheduling simulator, so only 1 process will be running at any giventime, and the scheduler needs to make a decision when the job finishes or when the job needs to be preempted. Theoutput from the simulator will be a simple sequence of the scheduled processes at each time step of the simulation,along with a final table of statistics with finish time, turnaround time (Tr) and ratio of turnaround time to servicetime (Tr/Ts).A working fist come first server (FCFS) scheduler has been given to you already in this assignment. You will beasked to implement one of the other scheduling policies. You can choose to implement a round robin scheduler (RR),shortest process next (SPN) shortest remaining time (SRT), highest response ratio next (HRRN), or a feedbackscheduler (FB).Questions• How is process scheduling accomplished in the OS.• What are the similarities in implementation between different process scheduling policies? What are theirdifferences?• What information does a scheduling algorithm need in order to select the next job to run on the system.• What are the differences between preemptive and non-preemptive scheduling policies?• How does the FCFS policy work? How do the other process scheduling policies we discuss work?• How do the various process scheduling policies compare in terms of performance? How do we measure good orbad performance for a process scheduler?Objectives• Implement a process scheduling policy using the process scheduler framework for this assignment.• Look at use of C++ virtual classes and virtual class functions for defining an interface/API• Better understand how process scheduling works in real operating systems, and in particular what informationis needed to make decisions on when to preempt and which process to schedule next.IntroductionIn this assignment you will be implementing some of the process schedulers that are discussed in our textbookchapter 9. As with the previous assignment, your first task will be to implement some of the missing pieces of thegeneral process scheduling simulator framework, that supports plugging in different job scheduling policies to makedispatching decisions. The simulator will take a table of job arrival information along with the service time (runtime) of the jobs, just as is used in our textbook for discussing the various processes scheduling policies. We will besimulating a single CPU system with this process scheduling simulator, so only 1 process will be running at any giventime, and the scheduler needs to make a decision when the job finishes or when the job needs to be preempted. Theoutput from the simulator will be a simple sequence of the scheduled processes at each time step of the simulation,1along with a final tabla of statistics with finish time, turnaround time (Tr) and ratio of turnaround time to servicetime (Tr/Ts).The process scheduler simulator framework consists of the following classes. There is a single class given in theSchedulingSystem[.hpp|cpp] source files that defines the framework of the process scheduler policy simulation.This class handles the outline of the simulation framework needed to simulate process scheduling in an operatingsystem. The class has methods to support loading files of job arrival and service time information, or to generaterandom job arrival tables. This class has a runSimulation() function that is the main hook into the simulator. Thebasic algorithm of the process scheduling simulator is to simulate time steps occurring in discrete time steps. Ateach time step the scheduling simulator framework determines if a new process arrives, if the current running processhas finished, and/or if the current running process should be preempted. All of these events are communicated tothe scheduler class, so that it may update its data structures (like ready queues or other information about waitingprocesses). If the cpu is idle at the beginning of the time step, it asks the scheduler class to make a process schedulingdecision, to select a process to begin running on the system.A separate abstract API/base class hierarchy is defined, using the Strategy design pattern, to implement the actualmechanisms for a process scheduling policy. The base class is named SchedulingPolicy.[cpp|hpp]. Most of thefunctions of this class are pure virtual functions, they describe an interface that is used by the SchedulingSystemclass to interact with a particular process scheduling policy. The first come first serve (FCFS) policy has beenimplemented already in this assignment, FCFSSchedulingPolicy[.cpp|hpp]. You may use this class as a referencewhen developing a new scheduling policy to add to the framework.Unit Test TasksFor this assignment, you will first of all be completing some of the functions in the SchedulingSystem class to getthe basic simulator running. Then once the simulator is complete, you will be implementing one of the remainingSchedulingPolicy to add capabilities to the system.So for this assignment, as usual, you should start by getting all of the unit tests to pass, and I strongly suggest youwork on implementing the functions and passing the tests in the given order. To get the simulator class completedyou will first need to complete the following tasks.
The first test case of the unit tests this week tests a few accessor methods that you need to implement. Thesemethods are found in the SchedulingSystem class. As a warm up exercise, and to get familiar with this class,start by completing the following accessor methods: getSystemTime(), getNumProcesses(), isCpuIdle(),getRunningProcessName().
The allProcessesDone() function is important for controlling when the simulation ends. You need to implementthis function. as well to get the first unit tests to completely pass. For this function, you need to search theprocess table. If you find a process that is not yet done (example the done member field for each Process),then the answer is false, not all processes are done. But if all of them are done, you should return true.
The runSimulation() function, as usual, is the hook into the simulation class to run a whole system simulation.The first function of the simulation, checkProcessArrivals() has been given already. It shows an example ofsending a message to the policy instance to notify it when new processes arrive. However, the next functiondispatchCpuIfIdle() has not yet been implemented. If the cpu is idle (use isCpuIdle() to test this), thenwe need to work with our policy to make a dispatching system. The policy instance has a method that, whencalled, will return a Pid which will be the process identifier of the process that should next be “dispatched”to run on the cpu. So if the cpu is idle, you should queary the policy object in this function to return theidentifier of the process to run next. Once you have the pid, you should set the cpu member variable to be thispid, which is equivalent to starting the process running on the cpu in thi system. You also need to record thestart time for the process. If the process has never run before, its startTime will be NOT_STARTED. If the processis running for the first time, make sure you record the current time as the processes startTime.
The simulateCpuCycle() function has been given to you, but you should take a look at it and understand itat this point. To simulate a cpu cycle, we simply increment the time used of the currently running process.We also record the schedule history of which process runs given this the process that is currently running.However, the next process checkProcessFinished() needs to be implemented. If the cpu is currently IDLEthen there is nothing to do, and you should return immediately (look at the next checkProcessPreemption()for an example). But if a process is currently running, check the current running process to see if its timeUsed2is equal to or exceeds the serviceTime for the process. If you look back at the simulateCpuCycle() method,you will see that timeUsed() is incremented for a process each cycle it is running on the cpu. So in thecheckProcessFinished() function, you can test if the timeUsed has reached the serviceTime. When theprocess is finished, you need to perform 3 tasks.a. Record the endTime for the running process, as it is now finished.b. Most important, set the process to be done. Each Process has a member variable named done that willbe initially false. When all processes are marked as done, then the simulation is finished.c. Also very important, the cpu should now be IDLE. You should set the simulator cpu member variable tothe IDLE value, so that a new process will be dispatched the next time it is checked to see if the cpu iscurrently idle or not.Once these 4 tasks are complete, all of the unit tests you were given should now be passing. The runSimulation()function has been implemented for you, and it will call the dispatchCpuIfIdle() and checkProcessFinished()functions you implemented (as well as need to use the getter methods from step 1).The next step is a bit more open ended. The simulation and unit tests you were given will use theFCFSSchedulingPolicy object by default to perform the tests using a first come first server (FCFS) schedulingpolicy. In the second part of the assignment, you are to implement 1 additional scheduling policy and add it to thesimulator. You can choose any of the simulation policies from chapter 9 (besides FCSF), such as a round robin (RR)scheduler, shortest process next (SPN), shortest remaining time (SRT), highest response ratio (HRRN) or a feedbackscheduler (FB). You should start by copying the files names FCFSSchedulingPolicy.[hpp|cpp] and renaming thefile name replacing FCFS with the mnemonic for the scheduling policy you will implement. You should also renamethe class names inside of the files as first step towards implementing them.You will then need to modify the class to implement your given strategy. You can and may need to use STL containersfor your policy class. For example, the FCFS class uses a standard STL queue instance to represent its ready queue.If you were to choose round robin, for example, you would also need a ready queue, but you would have to add in abit of extra mechanism to keep track of the running processes being assigned and using its time slice quantum, and topreempt the process when it time slice quantum has been used up, returning it to your ready queue.System Tests: Putting it all TogetherOnce all of the unit tests are passing, you can begin working on the system tests.As with the previous assignment, the assg05-sim.cpp creates a sim program that uses command line argument toset up and run a simulation. The paging system simulator is invoked like this:$ ./simUsage: sim policy process-table.sim [quantum]Run scheduling system simulation. The schedulingsimulator reads in a table of process information, specifyingarrival times and service times of processes to simulate.This program simulates the indicated process schedulingpolicy. Simulation is run until all processes are finished.Final data are displayed about the scheduling historyof which process ran at each time step, and the statisticsof the performance of the scheduling policy.Options:policy The page job scheduling policy to use, current‘FCFS’, ‘RR’, ‘SPN’ are valid and supportedprocess-table.sim Filename of process table information toto be used for the simulation.[quantum] Time slice quantum, only used by some policiesthat perform round-robin time slicingThe simulator requires 2 command line arguments to run, and a 3rd optional parameter. You first specify the policy,such as FCFS or RR, and then the location of the process table simulation file. For policies that use time slicing, thesystem time quantum can/should be specified as well.3Assignment SubmissionIn order to document your work and have a definitive version you would like to grade, a MyLeoOnline submissionfolder has been created named Assignment-04 for this assignment. There is a target in your Makefile for theseassignments named submit. When your code is at a point that you think it is ready to submit, run the submit target:$ make submittar cvfz assg05.tar.gz FCFSSchedulingPolicy.cpp RRSchedulingPolicy.cppSchedulingPolicy.cpp SchedulingSystem.cpp SPNSchedulingPolicy.cppFCFSSchedulingPolicy.hpp RRSchedulingPolicy.hpp SchedulingPolicy.hppSchedulingSystem.hpp SPNSchedulingPolicy.hppFCFSSchedulingPolicy.cppRRSchedulingPolicy.cppSchedulingPolicy.cppSchedulingSystem.cppSPNSchedulingPolicy.cppFCFSSchedulingPolicy.hppRRSchedulingPolicy.hppSchedulingPolicy.hppSchedulingSystem.hppSPNSchedulingPolicy.hppThe result of this target is a tared and gziped (compressed) archive, named assg05.tar.gz for this assignment. Youshould upload this file archive to the submission folder to complete this assignment. I will probably be also directlylogging into your development server, to check out your work. But the submission of the files serves as documentationof your work, and as a checkpoint in case you keep making changes that might break something from when you had itworking initially.Requirements and Grading RubricsProgram Execution, Output and Functional Requirements
Your program must compile, run and produce some sort of output to be graded. 0 if not satisfied.
10 pts all accessor methods from step 1 implemented and first test case passes.
10 pts the allProcessesDone() member function is implemented and working correctly.
20 pts checkProcessArrivals() implemented and working, test cases using this function are passing.
20 pts checkProcessFinished() implemented and working. Test cases using this function are passing.
40 pts Implemented a new process scheduling policy class. Implementation is correct and working. Added unittests to demonstrate the policy. Added the policy to the system simulation.Program Style and DocumentationThis section is supplemental for the first assignment. If you use the VS Code editor as described for this class, part ofthe configuration is to automatically run the uncrustify code beautifier on your code files every time you save thefile. You can run this tool manually from the command line as follows:$ make beautifyuncrustify -c ../../config/.uncrustify.cfg –replace –no-backup *.hpp *.cppParsing: HypotheticalMachineSimulator.hpp as language CPPParsing: HypotheticalMachineSimulator.cpp as language CPPParsing: assg01-sim.cpp as language CPPParsing: assg01-tests.cpp as language CPPClass style guidelines have been defined for this class. The uncrustify.cfg file defines a particular code style, likeindentation, where to place opening and closing braces, whitespace around operators, etc. By running the beautifieron your files it reformats your code to conform to the defined class style guidelines. The beautifier may not be able tofix all style issues, so I might give comments to you about style issues to fix after looking at your code. But youshould pay attention to the formatting of the code style defined by this configuration file.4Another required element for class style is that code must be properly documented. Most importantly, all functionsand class member functions must have function documentation proceeding the function. These have been givento you for the first assignment, but you may need to provide these for future assignment. For example, the codedocumentation block for the first function you write for this assignment looks like this:/**
@brief initialize memory*
Initialize the contents of memory. Allocate array larget enough to
hold memory contents for the program. Record base and bounds
address for memory address translation. This memory function
dynamically allocates enough memory to hold the addresses for the
indicated begin and end memory ranges.*
@param memoryBaseAddress The int value for the base or beginning
address of the simulated memory address space for this
simulation.
@param memoryBoundsAddress The int value for the bounding address,
e.g. the maximum or upper valid address of the simulated memory
address space for this simulation.*
@exception Throws SimulatorException if
address space is invalid. Currently we support only 4 digit
opcodes XYYY, where the 3 digit YYY specifies a reference
address. Thus we can only address memory from 000 – 999
given the limits of the expected opcode format.*/This is an example of a doxygen formatted code documentation comment. The two ** starting the block commentare required for doxygen to recognize this as a documentation comment. The @brief, @param, @exception etc. tagsare used by doxygen to build reference documentation from your code. You can build the documentation using themake docs build target, though it does require you to have doxygen tools installed on your system to work.$ make docsdoxygen ../../config/Doxyfile 2>&1| grep warning| grep -v “file statement”| grep -v “pagebreak”| sort -t: -k2 -n| sed -e “s|/home/dash/repos/csci430-os-sims/assg/assg01/||g”The result of this is two new subdirectories in your current directory named html and latex. You can use a regularbrowser to browse the html based documentation in the html directory. You will need latex tools installed to buildthe pdf reference manual in the latex directory.You can use the make docs to see if you are missing any required function documentation or tags in your documentation.For example, if you remove one of the @param tags from the above function documentation, and run the docs, youwould see$ make docsdoxygen ../../config/Doxyfile 2>&1| grep warning| grep -v “file statement”| grep -v “pagebreak”| sort -t: -k2 -n| sed -e “s|/home/dash/repos/csci430-os-sims/assg/assg01/||g”HypotheticalMachineSimulator.hpp:88: warning: The following parameter ofHypotheticalMachineSimulator::initializeMemory(int memoryBaseAddress,int memoryBoundsAddress) is not documented:5parameter ‘memoryBoundsAddress’The documentation generator expects that there is a description, and that all input parameters and return valuesare documented for all functions, among other things. You can run the documentation generation to see if you aremissing any required documentation in you project files.6
Get professional assignment help cheaply
Are you busy and do not have time to handle your assignment? Are you scared that your paper will not make the grade? Do you have responsibilities that may hinder you from turning in your assignment on time? Are you tired and can barely handle your assignment? Are your grades inconsistent?
Whichever your reason may is, it is valid! You can get professional academic help from our service at affordable rates. We have a team of professional academic writers who can handle all your assignments.
Our essay writers are graduates with diplomas, bachelor, masters, Ph.D., and doctorate degrees in various subjects. The minimum requirement to be an essay writer with our essay writing service is to have a college diploma. When assigning your order, we match the paper subject with the area of specialization of the writer.
Why choose our academic writing service?
Plagiarism free papers
Timely delivery
Any deadline
Skilled, Experienced Native English Writers
Subject-relevant academic writer
Adherence to paper instructions
Ability to tackle bulk assignments
Reasonable prices
24/7 Customer Support
Get superb grades consistently
Get Professional Assignment Help Cheaply
Are you busy and do not have time to handle your assignment? Are you scared that your paper will not make the grade? Do you have responsibilities that may hinder you from turning in your assignment on time? Are you tired and can barely handle your assignment? Are your grades inconsistent?
Whichever your reason may is, it is valid! You can get professional academic help from our service at affordable rates. We have a team of professional academic writers who can handle all your assignments.
Our essay writers are graduates with diplomas, bachelor’s, masters, Ph.D., and doctorate degrees in various subjects. The minimum requirement to be an essay writer with our essay writing service is to have a college diploma. When assigning your order, we match the paper subject with the area of specialization of the writer.
Why Choose Our Academic Writing Service?
Plagiarism free papers
Timely delivery
Any deadline
Skilled, Experienced Native English Writers
Subject-relevant academic writer
Adherence to paper instructions
Ability to tackle bulk assignments
Reasonable prices
24/7 Customer Support
Get superb grades consistently
How It Works
1. Place an order
You fill all the paper instructions in the order form. Make sure you include all the helpful materials so that our academic writers can deliver the perfect paper. It will also help to eliminate unnecessary revisions.
2. Pay for the order
Proceed to pay for the paper so that it can be assigned to one of our expert academic writers. The paper subject is matched with the writer’s area of specialization.
3. Track the progress
You communicate with the writer and know about the progress of the paper. The client can ask the writer for drafts of the paper. The client can upload extra material and include additional instructions from the lecturer. Receive a paper.
4. Download the paper
The paper is sent to your email and uploaded to your personal account. You also get a plagiarism report attached to your paper.
PLACE THIS ORDER OR A SIMILAR ORDER WITH Essay fount TODAY AND GET AN AMAZING DISCOUNT
The post introduction to operating systems on businesses appeared first on Essay fount.
What Students Are Saying About Us
.......... Customer ID: 12*** | Rating: ⭐⭐⭐⭐⭐"Honestly, I was afraid to send my paper to you, but you proved you are a trustworthy service. My essay was done in less than a day, and I received a brilliant piece. I didn’t even believe it was my essay at first 🙂 Great job, thank you!"
.......... Customer ID: 11***| Rating: ⭐⭐⭐⭐⭐
"This company is the best there is. They saved me so many times, I cannot even keep count. Now I recommend it to all my friends, and none of them have complained about it. The writers here are excellent."
"Order a custom Paper on Similar Assignment at essayfount.com! No Plagiarism! Enjoy 20% Discount!"
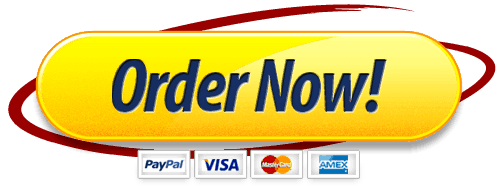